[sourcecode language="javascript"]
" this has padded whitespace ".trim();
[/sourcecode]
press Enter, and behold:
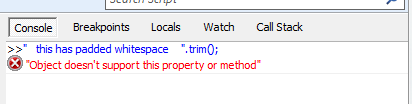
Insane! Thankfully, the folks at jQuery have taken care of this.
From Mvc2.TaskUI by Jonathan Oliver.
Array.prototype
, which I rolled into a file I call iterable.js:reduce
function. It’s not here because I have not needed it in my project yet, nor am I likely to. But it would be easy to implement:HashSet
class says, “The HashSet(Of T) class provides high performance set operations. A set is a collection that contains no duplicate elements, and whose elements are in no particular order.” Fair enough, except that the HashSet
class preserves insertion order. Furthermore, set1.Equals(set2)
will return false
even when the two sets contain the same elements but in a different insertion order.ICollection<T>
or something for more genericity.
I have many interests; one of them is linguistics. I first heard of the Sapir-Whorf hypothesis, also called the linguistic relativity principle, some years ago and it caught my interest. Despite the many objections and resistance brought against it, I have always found it to be rather compelling.
It seems obvious to me that the language we use to describe and interface with the world should shape our cognition about the world. Many studies have been done which show this in natural languages. But it seems that there have been fewer studies about this principle in programming languages, especially in software engineering.
I remember when I took CS 330 about a year ago. CS 330 is a course on programming languages, and the language of the class is Scheme, a cousin of Lisp. Scheme is a functional programming language, which is to say that, in Scheme, functions are first-class objects, just like any other data type. This was the first time I was ever really exposed to the functional paradigm (although I saw a little of it before in Javascript), and it had a rather profound effect on my skills as a software developer. For when we studied Scheme and used it to investigate the principles of programming languages, my thinking about programming changed; it expanded into new realms, and I could see things that I had never really seen before.
One of the things studying Scheme did for me was to get me interested in programming languages and related concepts. But more immediately, it added to my cognitive landscape the idea of functional programming. Not long after I began to learn Scheme did I come to see the benefits of functional programming in other languages, like Python, which I learned has a "lambda" keyword like Scheme. Soon I began to incorporate functional principles in my work outside that class, in other classes, and so on. I was writing software in a way that I would not have done before without having been exposed to this new paradigm.
And thus it is with software engineering. The long use of the same tools, practices, patterns, languages, etc. often constrain our thinking and deprive us from creating elegant, simple solutions to problems. While I am a firm believer in process---process in the sense that there must be some means by which a software development organization develops its software, and that it should be at least somewhat structured, I also acknowledge the fact that adhering too rigidly to process and procedure can stifle creativity and ossify the mentality of an organization. This is to be avoided, since software development is a creative enterprise; it is by no means formulaic.
We software engineers, programmers, or whatever we like to be called, are, in a sense, linguists. We learn and use languages all the time. As such, we are subject to linguistic principles. Despite the objections of Chomsky and others, I have always found Sapir-Whorf to be true---so true, in fact, that it's a self-evident proposition.